Imagine a world where deploying and running powerful applications is as simple as writing JavaScript, a world where infrastructure fades into the background, and your code just runs exactly where it needs to be: at the edge of the internet, right next to your users.
We're excited to announce that this world is now a reality and introduce Bunny Edge Scripting!
Edge Scripting is a new, serverless JavaScript platform built on top of Deno, designed to help developers build, deploy, and run JavaScript applications on our massive global network without worrying about hardware, scaling, or load balancing ever again.
With just a few clicks, Edge Scripting allows you to connect your GitHub repository and automatically deploy and run your application at the edge of the internet to enjoy unparalleled ease, speed, and scalability by simply pushing your code.
Connect. Push. Done.
When we designed Edge Scripting, our goal was simple: allow developers to build and run their applications in the simplest way possible, and with Bunny Edge Scripting, your GitHub main branch becomes your launchpad.
Connecting your account takes just a few clicks. Then, once you're done with your code changes, just push them to your main branch, and in just seconds, your app is built and deployed globally.
No more tedious compiling, versioning, rollback worries, or complex CI/CD configurations. Edge Scripting handles the heavy lifting so you can focus on what you do best: writing code. With automatic deployments and seamless integration into your development workflow, getting your application live has never been easier. It's as simple as connecting your repo, pushing your code, and you're live.
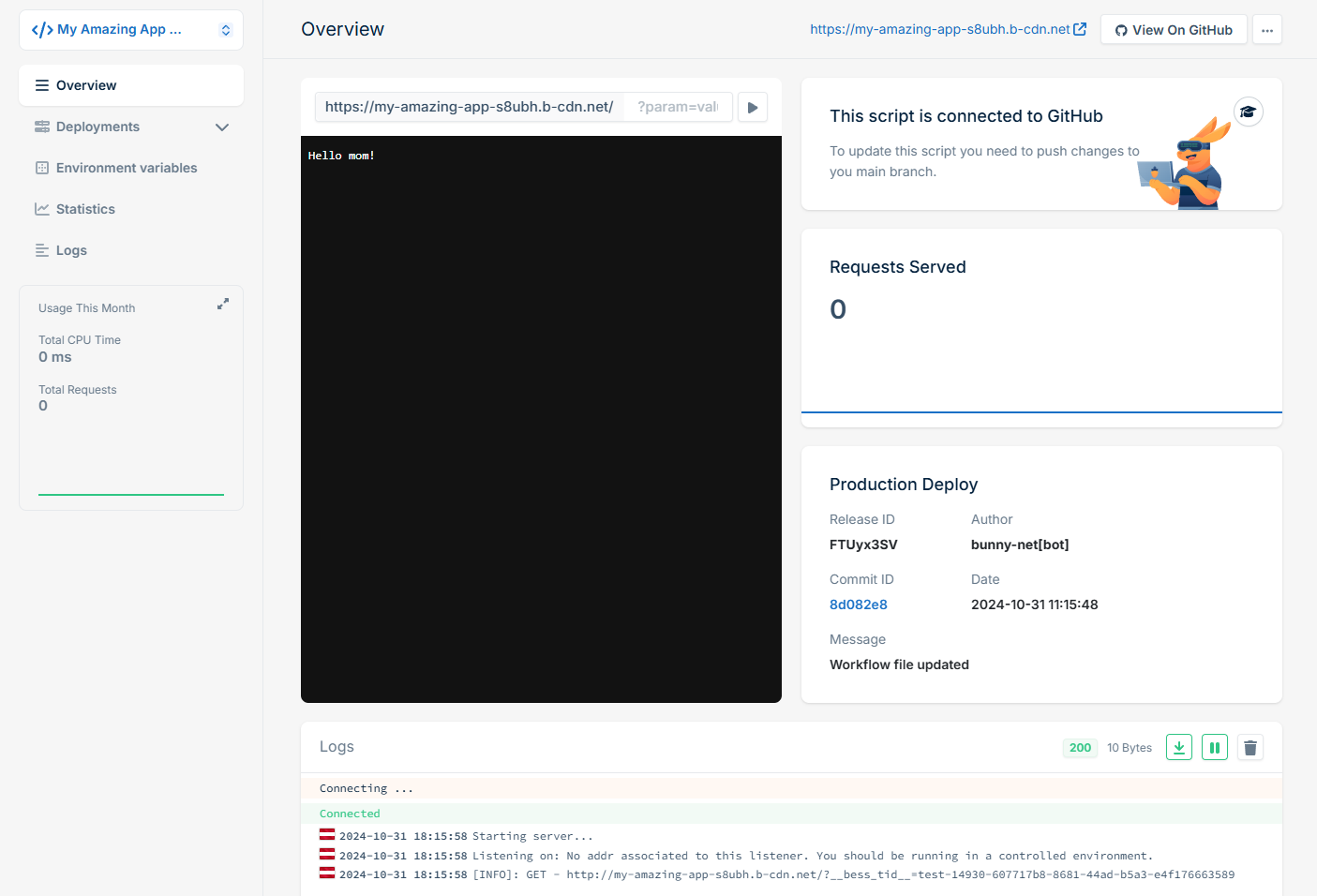
Infinite scalability without the hassle or the price tag
We've all been there: your app gains popularity, and suddenly, you're scrambling to add new servers. This instantly brings a mountain of new responsibilities, from monitoring, failover, load balancing, and security. All of this requires expertise, but more than anything, it requires time. The cloud was supposed to simplify this, but nowadays, it often seems to add even more complexity.
This is why we designed Edge Scripting to be as hands-free as possible. We've built it directly into our global network with automatic scalability, load balancing, failover, and robust security built-in without you even lifting a finger. Everything is seamlessly handled at the edge of the network.
What also helps you scale infinitely is the pricing.
We're on a mission to help make the internet hop faster, which also includes making it affordable to everyone. We believe that powerful compute resources should be accessible without breaking the bank. No matter if you're just building a simple contact form or running a massive global operation, Edge Scripting pricing was designed to work and scale with you.
It comes with no minimums, no complex billing, and no hidden costs. You only pay for the resources that you use:
- Requests: $0.2 per million
- CPU Time: $0.02 per 1,000 of CPU time seconds
If you're serving nothing? You're paying nothing, too!
Make the CDN edge your playground with Middleware Apps
Edge Scripting wasn't just a compute product that we bolted on and deployed globally. Instead, we built it straight into the fabric of one of the largest networks on the internet. As a result, Edge Scripting turns our ultra-fast global network into a CDN that you can program yourself. With this, we're unlocking powerful new ways to build, optimize, and secure applications closer to users than ever before.
Today, bunny.net is an entry point for over 1.5 million websites and moreover, serves assets for millions of other domains. This gives us a powerful role in security, load balancing, caching, and smart logic at the edge. While we already offer powerful features such as Edge Rules, Edge Scripting takes this to a whole new level, making the edge your playground with the new Middleware Apps.
Middleware Apps were designed to go beyond standalone applications and provide a toolkit for customizing the CDN behavior on a much deeper level. With Middleware Apps, you can integrate directly into Bunny CDN and seamlessly extend it with your own functionality.
Be it load balancing, security checks, A/B testing, caching, or request modification, the Middleware mode allows you to take control of how the CDN should respond and adjust it on demand. It’s as close as you can get to running your own custom CDN but without the infrastructure overhead.
At launch, Middleware Apps support modifying the origin request and response, but this is only the beginning. We are actively working on expanding this functionality to give you even deeper control over caching, revalidation, and other core CDN behaviors soon.
Your first edge application takes just a few lines of code
Edge Scripting isn't just simple to deploy, it's also incredibly easy to code. To help you effortlessly run standalone applications across Deno, Node.js, and Bunny Edge Scripting, we created BunnySDK. The SDK is a lightweight wrapper that closely mirrors the Deno server implementation but adds the ability to run seamlessly across different environments.
This makes getting started with Edge Scripting unbelievably simple, taking as little as a few lines of code.
Hello World example:import * as BunnySDK from "https://esm.sh/@bunny.net/edgescript-sdk@0.11.2"; /** * Returns an HTTP response. * @param {Request} request - The Fetch API Request object. * @return {Response} The HTTP response or string. */ BunnySDK.net.http.serve(async (request: Request): Response | Promise<Response> => { return new Response("Hello World"); });
The snippet above is all it takes to create a global, edge-optimized application that is able to respond instantly to user requests at the edge, but also run locally on your machine.
Middleware Applications are equally simple. We've made it extremely easy to modify both the request and response of any CDN request. Middleware Apps allow you to inject custom logic directly into the existing CDN processing pipeline. This provides a seamless integration into any origin configuration, Edge Rules, and Pull Zone configuration without having to rewrite any complex proxy logic yourself.
Middleware App example:import * as BunnySDK from "https://esm.sh/@bunny.net/edgescript-sdk@0.11.2"; /** * When a response is not served from the cache, you can use this event handler * to modify the request going to the origin. * * @param {Context} context - The context of the middleware. * @param {Request} context.request - The current request. */ async function onOriginRequest(context: { request: Request }): Promise<Response> | Response | Promise<Request> | Request | void { context.request.headers.append("Before", "Added before request"); } /** * When a response is not served from the cache, you can use this event handler * to modify the response going from the origin. * This modifies the response before being cached. * * Returns an HTTP response. * @param {Context} context - The context of the middleware. * @param {Request} request - The current request done to the origin. * @param {Response} response - The HTTP response or string. */ async function onOriginResponse(context: { request: Request, response: Response }): Promise<Response> | Response | void { context.response.headers.append("After", "Added on the response return."); } BunnySDK.net.http.servePullZone() .onOriginRequest(onOriginRequest) .onOriginResponse(onOriginResponse);
It's this easy to add a request and response header to any Pull Zone. You can then easily trigger the script for all the requests or even execute dynamically using Edge Rules.
Of course, writing software is not just about producing code, but sharing it. One of our reasons for building Edge Scripting on top of Deno was its existing support for a vast ecosystem of external packages. For example, integrating an external service like SendGrid to send an email is this simple:
SendGrid example:import * as BunnySDK from "@bunny.net/edgescript-sdk"; import sgMail from "https://esm.sh/@sendgrid/mail@7.7.0"; // Set your SendGrid API key sgMail.setApiKey(Deno.env.get('SENDGRID_API_KEY')); BunnySDK.net.http.serve(async (req) => { const msg = { to: 'user@example.com', from: 'you@yourdomain.com', subject: 'Welcome to Bunny Edge Scripting!', text: 'This email was sent directly from the edge.', }; try { await sgMail.send(msg); return new Response("Email sent successfully!"); } catch (error) { console.error(error); return new Response("Failed to send email.", { status: 500 }); } });
Gone are the days of wasting hours to build, deploy, and connect a simple contact form. Now, all it takes is a few lines of code.
To learn how to get started easily and for more examples, you can visit the Edge Scripting documentation.
Powered by Deno: Flexibility, speed, and freedom without the lock-in
When we set out to build Bunny Edge Scripting, we set out to reinvent the user experience for how developers build, deploy, and run applications at the edge. What we didn't want to do was to build yet another JavaScript runtime with limited capabilities. We wanted developers to be able to build on a familiar ecosystem, complete with millions of modules, and without the burden of lock-in.
That's why we decided to combine the speed of bunnies with the might of dinosaurs, and build Edge Scripting on top of Deno. Deno is an open-source, modern JavaScript runtime designed to uncomplicate JavaScript development and eliminate unnecessary complexity. Best to be said by Ryan, the Deno founder himself:
On top of just being simple, building on Deno brings a list of additional advantages:
- Native TypeScript support: You can easily write type-safe code in TypeScript without any extra configuration.
- Enhanced security: Deno runs in a secure sandbox by default, minimizing vulnerabilities.
- Ultra-fast performance: Being built in Rust, Deno offers exceptional performance and ultra-low startup times.
- A vast ecosystem of modules: Deno supports tens of thousands of native modules and millions of npm packages to help you build anything easily.
We're excited to empower developers with a platform that doesn't focus on locking you in, but instead, empowers you to easily build and run your applications anywhere.
Batteries included: Compute, performance, and security
Out of the box, Deno comes with everything you need to build, test, and deploy your applications. With Edge Scripting, we're building further on top of that.
Leveraging our massive global footprint, any application deployed to bunny.net is automatically accelerated by our global network and automatically integrates with edge caching, powerful security, logging, and analytics.
This means your applications are always protected by powerful DDoS protection, application attacks, and have built-in CDN resource caching for the absolute best performance possible.
There's no more need to combine multiple different services for DNS, CDN, and load balancing. With Edge Scripting, it all comes built-in by default. On top of that, Edge Scripting adds even more crucial features to help you run applications globally, such as real-time logging, release control, environmental variables, and secret management.
Built-in real-time logging
Debugging and logging on a globally distributed scale brings some unique challenges, and with thousands of servers across 119+ datacenters globally, we understand this very well.
To make these challenges as simple as possible for developers, we built Edge Scripting with built-in real-time logging, allowing you to seamlessly observe what your app is doing in the wild.
With the live log view, you can quickly see what's going on, and dig deeper into specific issues thanks to regional filters and search support.
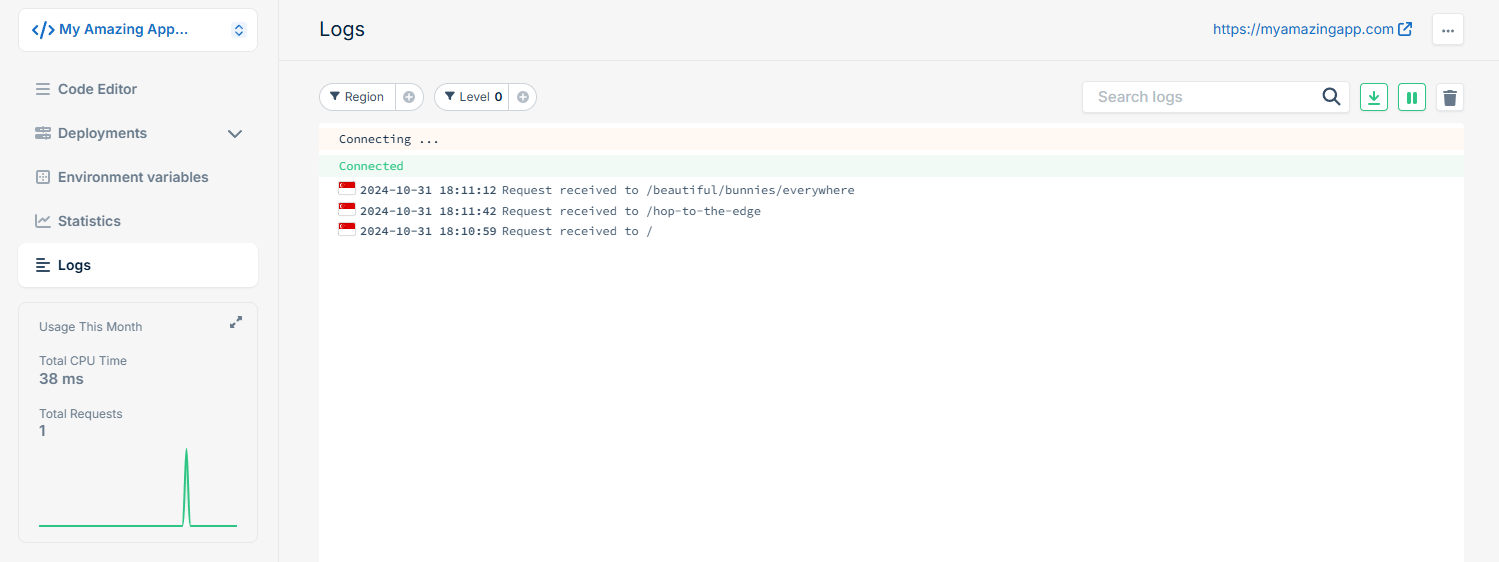
For advanced users, we also exposed the logging via an HTTP and a live WebSocket API, allowing you to easily connect it to other platforms.
Simple version and release controls
We get it, sometimes things break. A critical bug slips through or an unexpected rollback is urgently needed. That's why Edge Scripting introduces a simple, and intuitive version control system. Every time you make a change, or publish a release, we automatically create a version history for you, making instant global rollbacks just a few clicks away.
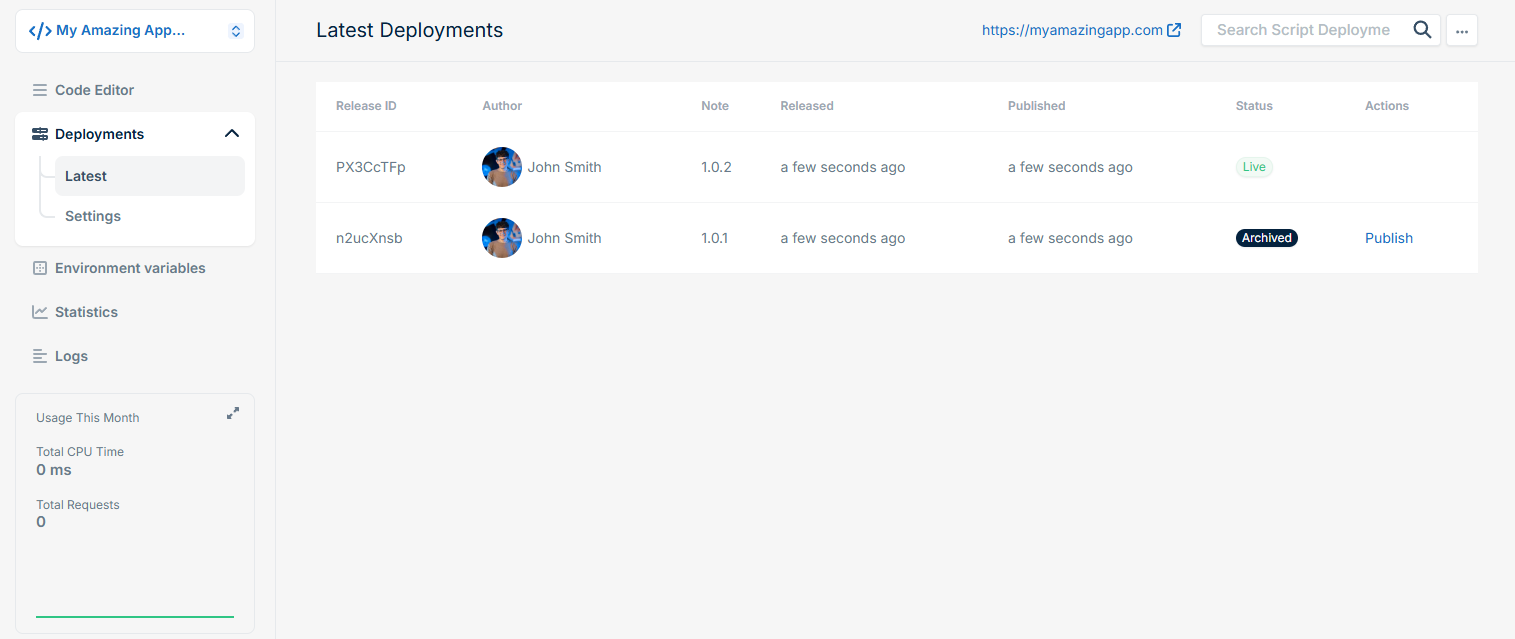
Even better, we are taking it a step further. Soon, we plan to introduce a built-in dynamic developer mode, allowing you to easily run a specific commit, release, or package using a dynamically mapped application URL. That means as soon as you push code, you can instantly test it live, without disrupting your production environment.
Full API and Terraform support
We understand that automation and infrastructure as code are essential for any modern development workflow. That's why, like all of our products, Bunny Edge Scripting comes with comprehensive API support as well as a Terraform provider.
This enables you to manage and deploy your applications programmatically and easily integrate into your existing CI/CD pipelines. With near-real-time configuration changes and deployments, Edge Scripting allows you to build powerful workflows, automate tasks, and execute dynamic code—giving you a robust automation tool with just a few API calls.
Moving beyond JavaScript with WASM support
Today, JavaScript is by far the most popular programming language in the world, but why stop there? For those wanting to do more, Deno also brings WebAssembly (WASM) support, allowing you to bring in Rust, C++, or any other language, and make it run globally with just as much ease. This provides even faster and more consistent performance, rivaling low-level native code.
Getting WASM running is very straightforward, and Deno conveniently exposes WebAssembly to the standard browser interface. All you need is a binary to run.
WASM counter example:import * as BunnySDK from "https://esm.sh/@bunny.net/edgescript-sdk@0.11.2"; const { instance, module } = await WebAssembly.instantiateStreaming( fetch("https://wpt.live/wasm/incrementer.wasm"), ); /** * Returns an HTTP response. * @param {Request} request - The Fetch API Request object. * @return {Response} The HTTP response or string. */ BunnySDK.net.http.serve(async (request: Request): Response | Promise<Response> => { const increment = instance.exports.increment as (input: number) => number; return new Response("Value " + increment(41)); });
It's that easy to run almost any application globally. FFmpeg at the edge? Not a problem. Weaving your next Rust or C++ applications into the fabric of the internet? Not a problem either. WASM lets you move beyond the limits of a single programming language. You are free to build, deploy, and code practically anything.
For more details on WASM support, have a look at Deno's WASM documentation.
Live in the blink of an eye
In line with our mission to make the internet hop faster, Edge Scripting isn't just simple, it's also blazing fast!
Deno was written in Rust and crafted for ultra-low startup times and blazing-fast performance. Without heavy dependencies, a highly optimized I/O pipeline, and native TypeScript support, applications hosted on Edge Scripting get as close as possible to actual 0ms startup times.
With Bunny Edge Scripting, you get the kind of performance that’s as close to 0ms startup as it gets. We’ve cut out the bloat and fine-tuned every layer of the request pipeline so that your applications respond before your users can even blink. Because at the edge, every millisecond matters.
Pawing the future of edge: Building the best Edge Computing platform
We're setting out to make ultra-fast global applications a breeze, and for us, this is just the beginning. We are excited to share that we have a rich roadmap ahead to make Edge Scripting the best way for anyone to build, and run any type of application.
Here's what's coming soon:
- A globally distributed database
- bunny.net storage library
- Support for npm module resolves
- Better node specifier support
- GPU and AI inference support
- Support for larger applications
- On-the-fly dynamic builds based on each commit
- CLI support
- And much, much more to follow...
As we continue to evolve Bunny Edge Scripting, we're committed to pushing the boundaries of what's possible at the edge, and build an edge computing platform of every developer's dream.
Hop into the future of Edge Computing
At bunny.net, we're on a mission to make the internet hop faster, and what better way to achieve that than by moving your applications just milliseconds away from your users.
If you're tired of dealing with complicated, slow, and expensive infrastructure, and you want to embrace a future where deploying is as simple as writing code, we invite you to hop onto Edge Scripting and give it a try!
You can sign up, and apply for our beta program today, and start focusing on what you enjoy most: creating awesome software! We're excited to build a faster, safer, and more reliable internet together!